I've had simple auditing set up in my databases for years thanks to our old DBA. This is not robust transaction logging, it just answers the questions "Who created this?", "When was this created?", "Who last edited this?" and "When was this last edited?".
After witnessing a brand new table audit itself without triggers, I set about to understand the setup. This seemed curious to me, so I thought it might also be curious to you.
It turned out to be a two step system which rendered my insert triggers superfluous (at least I think so, tell me if I'm missing something and I need insert triggers after all).
Our solution uses a combination of two methods. For inserts we are going to tie our audit fields to User Defined Data Types which inherit Defaults we have specified. For updates we will build a trigger to log the changes. We will use the following fields in our tables to track inserts and updates:
STEP 1: Create Defaults
Defaults, according to the SQL Server Help, specify what values are used in a column if you do not specify a value for the column when inserting a row. Often we will manually specify a default (e.g. "0" for an integer field to hold counts)
In SQL Server, right-click on "Defaults" and select "New Default".
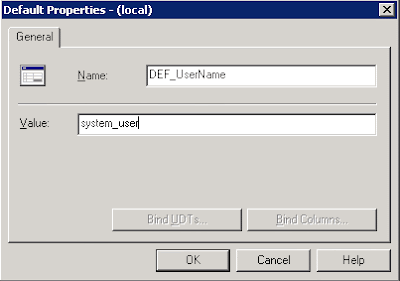
Default for logging Insert User
Default for logging Insert Date
NOTE: If the user is logged into SQL Server using Windows Authentication, SYSTEM_USER returns the Windows 2000 or Windows NT 4.0 login (e.g. "MYDOMAIN\myusername"). If the user is logged in to SQL Server using SQL Server Authentication, SYSTEM_USER returns the SQL Server login (e.g. "sa").
STEP 2: Create User-Defined Data Types
User-defined data types allow one to build custom specifications beyond the generic integer, decimal, varchar options. For example, one could create a custom character field of length 5 to store zip codes.
In SQL Server, right-click on "User Defined Data Types" and select "New User Defined Data Type".
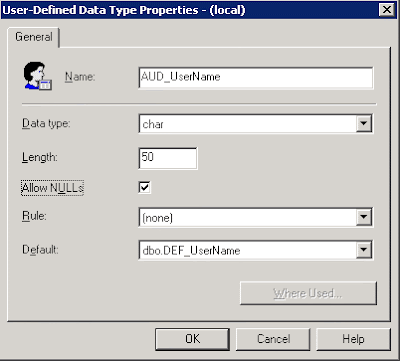
User-Defined Data Type for storing Usernames
User-Defined Data Type for storing Timestamps
STEP 3: Creating Audit Fields in the Database Table
Add the following audit fields to your database table. Set the Data Types to your new User Defined Types, note the Length, Default Value and Allow Nulls fields will be configured automatically.
Congratulations, inserts are now covered! And the best part is, for any future table you only have to repeat Step 3 (we'll look into automating that too in a future post).
On to updates....
STEP 4: Create a Trigger to log Updates
In SQL Server, right-click on your a Table with Audit Fields and select "All Tasks" and then "Manage Triggers". The Trigger Properties dialog will open with "<new>" selected as the name. Below this in the "Text:" textbox enter the following substituting [tablename] and [primarykey] to match your table.
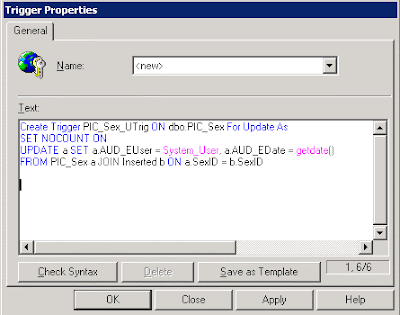
You now have basic auditing in your database that works no matter where the edits take place be in SQL Server, MS Access, a custom application or even ArcGIS.
Yes, ArcGIS! And not only when editing a linked SQL Server data table, but also with integrated security now part of ArcGIS, you can add these edit fields to an SDE Table using the same steps described above and log edits to an SDE feature layer.
After witnessing a brand new table audit itself without triggers, I set about to understand the setup. This seemed curious to me, so I thought it might also be curious to you.
It turned out to be a two step system which rendered my insert triggers superfluous (at least I think so, tell me if I'm missing something and I need insert triggers after all).
Our solution uses a combination of two methods. For inserts we are going to tie our audit fields to User Defined Data Types which inherit Defaults we have specified. For updates we will build a trigger to log the changes. We will use the following fields in our tables to track inserts and updates:
AUD_NUser - Creator
AUD_NDate - Creation Date
AUD_EUser - Editor
AUD_EDate - Edit Date
STEP 1: Create Defaults
Defaults, according to the SQL Server Help, specify what values are used in a column if you do not specify a value for the column when inserting a row. Often we will manually specify a default (e.g. "0" for an integer field to hold counts)
In SQL Server, right-click on "Defaults" and select "New Default".
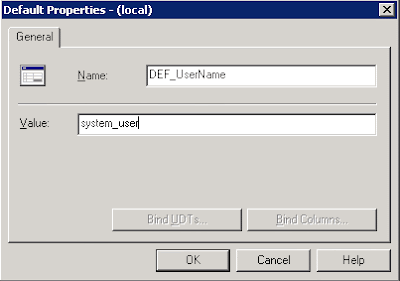
Name: "DEF_UserName"
Value: "system_user"
Name: "DEF_Date"
Value: "GetDate()"
NOTE: If the user is logged into SQL Server using Windows Authentication, SYSTEM_USER returns the Windows 2000 or Windows NT 4.0 login (e.g. "MYDOMAIN\myusername"). If the user is logged in to SQL Server using SQL Server Authentication, SYSTEM_USER returns the SQL Server login (e.g. "sa").
STEP 2: Create User-Defined Data Types
User-defined data types allow one to build custom specifications beyond the generic integer, decimal, varchar options. For example, one could create a custom character field of length 5 to store zip codes.
In SQL Server, right-click on "User Defined Data Types" and select "New User Defined Data Type".
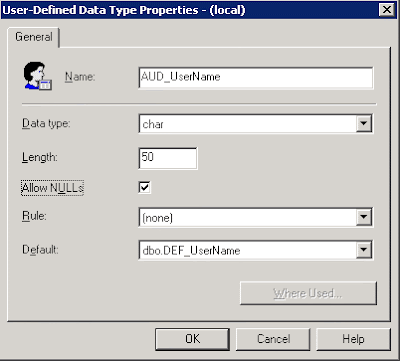
Name: "AUD_UserName"
Data type: "char"
Length: "50" (note this may need to be longer depending on your domain and usernames)
Allow NULLS: Check
Rule: "(none)"
Default: "dbo.DEF_UserName"
Name: "AUD_Timestamp"
Data type: "smalldatetime"
Length: "4"
Allow NULLS: Check
Rule: "(none)"
Default: "dbo.DEF_Date"
STEP 3: Creating Audit Fields in the Database Table
Add the following audit fields to your database table. Set the Data Types to your new User Defined Types, note the Length, Default Value and Allow Nulls fields will be configured automatically.
Field Data Type
AUD_NUser AUD_UserName
AUD_NDate AUD_Timestamp
AUD_EUser AUD_UserName
AUD_EDate AUD_Timestamp
Congratulations, inserts are now covered! And the best part is, for any future table you only have to repeat Step 3 (we'll look into automating that too in a future post).
On to updates....
STEP 4: Create a Trigger to log Updates
In SQL Server, right-click on your a Table with Audit Fields and select "All Tasks" and then "Manage Triggers". The Trigger Properties dialog will open with "<new>" selected as the name. Below this in the "Text:" textbox enter the following substituting [tablename] and [primarykey] to match your table.
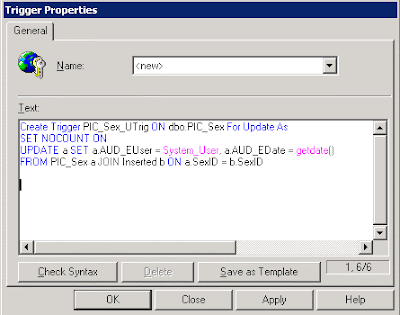
Create Trigger [tablename]_UTrig ON dbo.[tablename] For Update As
SET NOCOUNT ON
UPDATE a SET a.AUD_EUser = System_User, a.AUD_EDate = getdate()
FROM [tablename] a JOIN Inserted b ON a.[primarykey] = b.[primarykey]
You now have basic auditing in your database that works no matter where the edits take place be in SQL Server, MS Access, a custom application or even ArcGIS.
Yes, ArcGIS! And not only when editing a linked SQL Server data table, but also with integrated security now part of ArcGIS, you can add these edit fields to an SDE Table using the same steps described above and log edits to an SDE feature layer.
Comments
In repayment, I've asked my coworker if he could document the template he built to add similar logging functionality to ArcSDE. I'll get that posted as soon as he gets back to me. Should be sometime Wednesday, sorry!
If you got updates working you can accomplish the insert auditing with triggers as well!
Here's the code you'll need for the insert trigger:
CREATE Trigger [tablename]_ITrig ON dbo.[tablename] For Insert As
SET NOCOUNT ON
a.AUD_NUser = System_User, a.AUD_NDate = getdate(), a.AUD_EUser = System_User, a.AUD_EDate = getdate() FROM [tablename] a JOIN Inserted b ON a.[primarykey] = b.[primarykey]
Please let me know if you get this to work!